Subdivide mesh
- Peter Mortier
- 7 minutes ago
- 1 min read
Subdividing a mesh is the process of increasing its resolution by breaking down its elements into smaller parts. Subdivision adds more points and elements while preserving the overall shape.
In this example, we start with a basic triangular mesh consisting of a single triangle. Using the subdivide() function, we create two refined versions of the original triangle by dividing each edge into smaller segments.
In the first version, we subdivide the triangle so that each edge is split into 4 segments.
In the second version, we create a more detailed mesh where each edge is split into 10 segments.
This demonstrates how easily mesh resolution can be increased using the subdivide() function from the HelloTriangle library.
# generate basic triangle
points = [[0.0, 0.0, 0.0],
[1.0, 0.0, 0.0],
[0.0, 1.0, 0.0]]
conn = [[0, 1, 2]]
triangle = Mesh(points, conn, eltype='tri3')
# subdivide
triangleMed = triangle.subdivide(4)
triangleFine = triangle.subdivide(10)
# translate for visualization purposes
triangle = triangle.translate([-0.6, -0.6, 0.0])
triangleFine = triangleFine.translate([0.6, 0.6, 0.0])
# draw
draw(triangle, color = "#006992", show_edges = True)
draw(triangleMed, color = "#006992", show_edges = True)
draw(triangleFine, color = "#006992", show_edges = True)
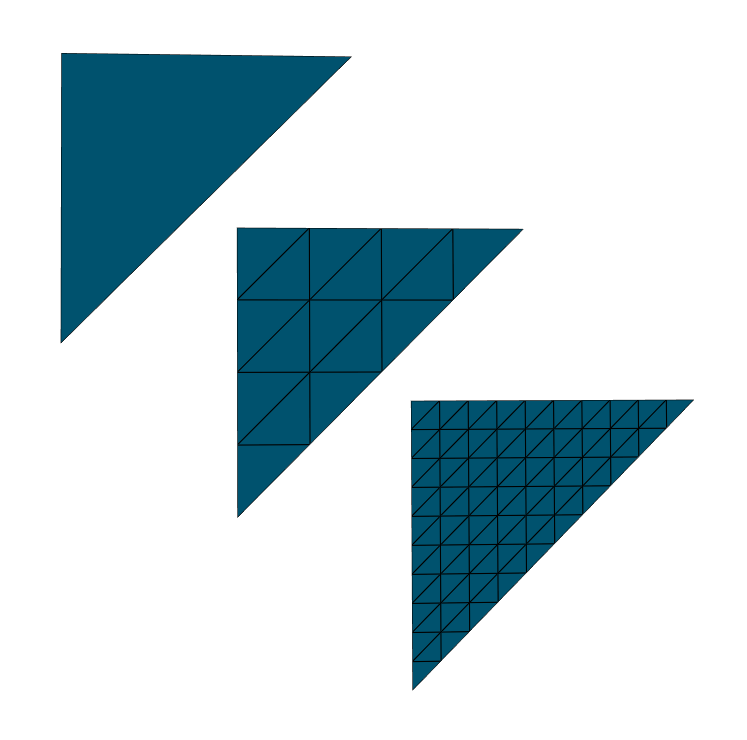
Below another short example in which the subdivision is being applied to a single hexahedral element.
from hellotriangle import shapes
# generate basic cube consisting of a single hex element
cube = shapes.cube(eltype='hex8')
# subdivide
cubeFine = cube.subdivide(10)
# translate original cube
cube = cube.translate([-1.0, -1.0, 1.0])
# draw
draw(cube, color = "#006992", show_edges = True)
draw(cubeFine, color = "#006992", show_edges = True)
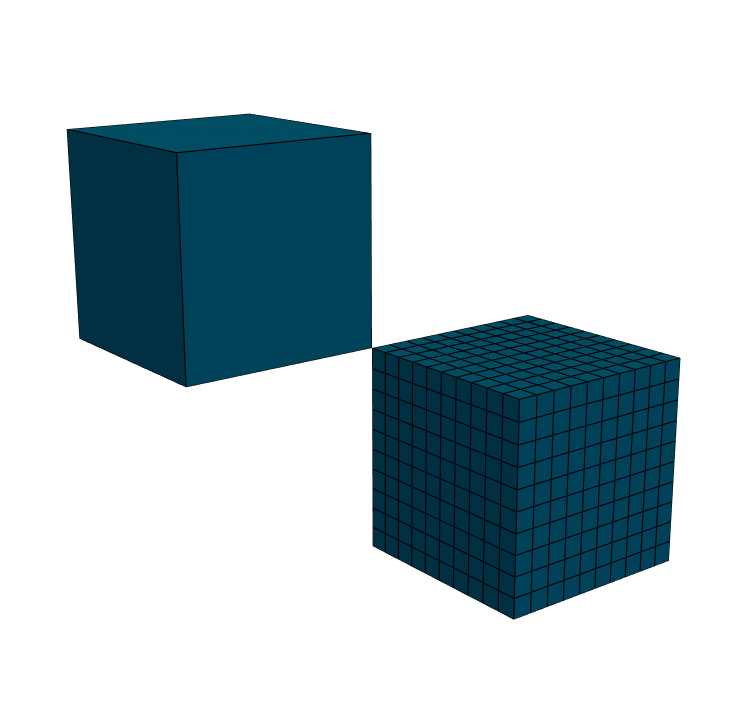